WordPress Action Hooks:
Learn how to use WordPress action hooks to customize your site’s functionality with our comprehensive beginner’s guide. Our guide includes 25 essential action hooks with example code to help you get started with customizing your WordPress site.
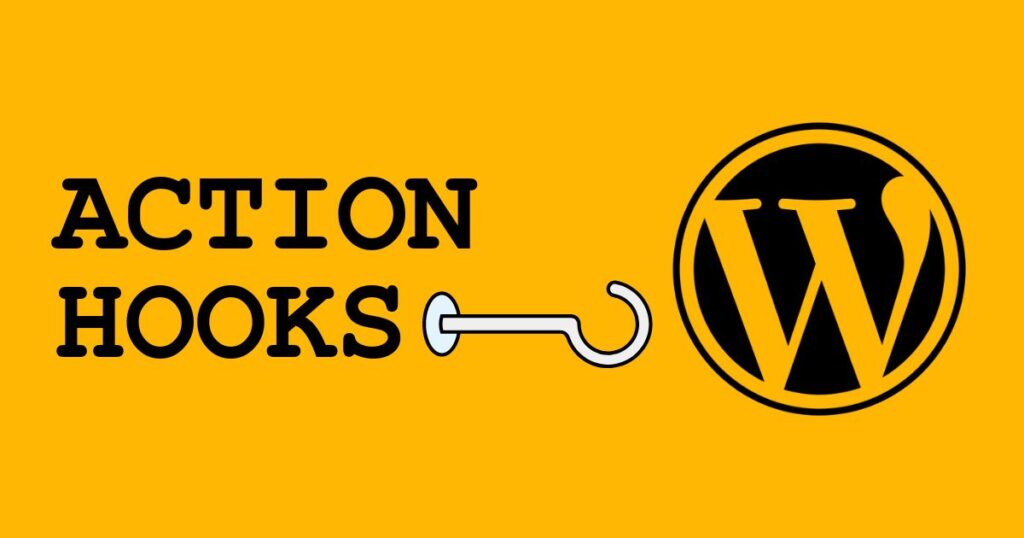
Action hooks are an essential part of WordPress development as they allow developers to execute their code at specific points during the execution of WordPress.
To use the WordPress action hooks, you can copy these code snippets and paste them into your theme’s ‘functions.php’ file. It is better to create a child theme instead of changing directly to the main theme. Or you can create a plugin to use these hooks via a Plugin.
1: init
This action hook is used to initialize WordPress and is executed before the page is loaded. This could be used to perform custom initialization tasks or to register custom post types or taxonomies.
function custom_init_function() {
// Code to execute during the init action
}
add_action( 'init', 'custom_init_function' );
2: wp_head
This action hook is located within the head section of the WordPress theme and is used to add additional content to the head section of the page. This could be used to add custom CSS or JavaScript files, as well as meta tags or other information that should be included in the head section of the page.
function custom_wp_head_function() {
echo '';
}
add_action( 'wp_head', 'custom_wp_head_function' );
3: wp_footer
This action hook is located within the footer section of the WordPress theme and is used to add additional content to the footer section of the page. This could be used to add custom JavaScript files or other scripts that need to be executed at the end of the page.
function custom_wp_footer_function() {
echo '';
}
add_action( 'wp_footer', 'custom_wp_footer_function' );
4: wp_enqueue_scripts
This action hook is used to enqueue scripts and styles in WordPress. This could be used to add custom JavaScript or CSS files to the site.
function custom_enqueue_scripts_function() {
wp_enqueue_style( 'custom-style', 'path/to/custom-style.css', array(), '1.0.0', 'all' );
wp_enqueue_script( 'custom-script', 'path/to/custom-script.js', array( 'jquery' ), '1.0.0', true );
}
add_action( 'wp_enqueue_scripts', 'custom_enqueue_scripts_function' );
5: admin_enqueue_scripts
This action hook is used to enqueue scripts and styles in the WordPress admin area. This could be used to add custom JavaScript or CSS files to the WordPress admin area.
function custom_admin_enqueue_scripts_function() {
wp_enqueue_style( 'custom-admin-style', 'path/to/custom-admin-style.css', array(), '1.0.0', 'all' );
wp_enqueue_script( 'custom-admin-script', 'path/to/custom-admin-script.js', array( 'jquery' ), '1.0.0', true );
}
add_action( 'admin_enqueue_scripts', 'custom_admin_enqueue_scripts_function' );
6: pre_get_posts
This action hook is used to modify the main query before it is executed. This could be used to modify the query parameters or to add custom meta queries.
function custom_pre_get_posts_function( $query ) {
if ( $query->is_main_query() ) {
$query->set( 'meta_key', 'custom-meta-key' );
$query->set( 'meta_value', 'custom-meta-value' );
}
}
add_action( 'pre_get_posts', 'custom_pre_get_posts_function' );
7: save_post
This action hook is used to execute code after a post is saved or updated. This could be used to update post meta data or to perform other actions when a post is saved.
function custom_save_post_function( $post_id ) {
// Code to execute after a post is saved or updated
}
add_action( 'save_post', 'custom_save_post_function' );
8: delete_post
This action hook is used to execute code after a post is deleted. This could be used to perform cleanup tasks or to remove associated meta data.
function custom_delete_post_function( $post_id ) {
// Code to execute after a post is deleted
}
add_action( 'delete_post', 'custom_delete_post_function' );
9: wp_insert_post
This action hook is used to execute code after a post is inserted into the database. This could be used to perform additional tasks when a post is created.
function custom_wp_insert_post_function( $post_id, $post, $update ) {
// Code to execute after a post is inserted into the database
}
add_action( 'wp_insert_post', 'custom_wp_insert_post_function', 10, 3 );
10: wp_insert_comment
This action hook is used to execute code after a comment is inserted into the database. This could be used to perform additional tasks when a comment is created.
function custom_wp_insert_comment_function( $comment_id, $comment, $comment_approved ) {
// Code to execute after a comment is inserted into the database
}
add_action( 'wp_insert_comment', 'custom_wp_insert_comment_function', 10, 3 );
11: wp_login
This action hook is used to execute code after a user logs in to WordPress. This could be used to perform additional tasks when a user logs in.
function custom_wp_login_function( $user_login, $user ) {
// Code to execute after a user logs in to WordPress
}
add_action( 'wp_login', 'custom_wp_login_function', 10, 2 );
12: wp_logout
This action hook is used to execute code after a user logs out of WordPress. This could be used to perform additional tasks when a user logs out.
function custom_wp_logout_function() {
// Code to execute after a user logs out of WordPress
}
add_action( 'wp_logout', 'custom_wp_logout_function' );
13: wp_dashboard_setup
This action hook is used to add custom widgets to the WordPress dashboard. This could be used to display custom data or information to users.
function custom_wp_dashboard_setup_function() {
wp_add_dashboard_widget( 'custom_dashboard_widget', 'Custom Widget Title', 'custom_dashboard_widget_function' );
}
add_action( 'wp_dashboard_setup', 'custom_wp_dashboard_setup_function' );
function custom_dashboard_widget_function() {
// Code to display custom data or information in the dashboard widget
}
14: wp_ajax_(action)
This action hook is used to handle AJAX requests in WordPress. This could be used to perform custom actions or retrieve data via AJAX.
function custom_wp_ajax_function() {
// Code to handle AJAX request
}
add_action( 'wp_ajax_custom_action', 'custom_wp_ajax_function' );
15: wp_print_scripts
This action hook is used to add custom scripts to the WordPress frontend. This could be used to add custom functionality or styles to the frontend.
function custom_wp_print_scripts_function() {
wp_enqueue_script( 'custom_script', 'https://example.com/custom-script.js' );
}
add_action( 'wp_print_scripts', 'custom_wp_print_scripts_function' );
16: wp_loaded
This action hook is used to execute code after WordPress has finished loading. This could be used to perform additional tasks after WordPress has finished loading.
function custom_wp_loaded_function() {
// Code to execute after WordPress has finished loading
}
add_action( 'wp_loaded', 'custom_wp_loaded_function' );
17: shutdown
This action hook is used to execute code when PHP shuts down. This could be used to perform cleanup tasks or to log errors.
function custom_shutdown_function() {
// Code to execute when PHP shuts down
}
add_action( 'shutdown', 'custom_shutdown_function' );
18: transition_post_status
This action hook is used to execute code when a post’s status changes. This could be used to perform additional tasks when a post is published, unpublished, or deleted.
function custom_transition_post_status_function( $new_status, $old_status, $post ) {
// Code to execute when post status changes
}
add_action( 'transition_post_status', 'custom_transition_post_status_function', 10, 3 );
19: admin_head
This action hook is used to add custom code to the head section of the WordPress admin area. This could be used to add custom meta tags or other information to the head section.
function custom_admin_head_function() {
echo '';
}
add_action( 'admin_head', 'custom_admin_head_function' );
20: admin_footer
This action hook is used to add custom code to the footer section of the WordPress admin area. This could be used to add custom scripts or other information to the footer section.
function custom_admin_footer_function() {
echo '';
}
add_action( 'admin_footer', 'custom_admin_footer_function' );
21: get_header
This action hook is used to execute code before the header is loaded on the front end of the website. This could be used to perform additional tasks before the header is loaded.
function custom_get_header_function() {
// Code to execute before header is loaded
}
add_action( 'get_header', 'custom_get_header_function' );
22: get_footer
This action hook is used to execute code before the footer is loaded on the front end of the website. This could be used to perform additional tasks before the footer is loaded.
function custom_get_footer_function() {
// Code to execute before footer is loaded
}
add_action( 'get_footer', 'custom_get_footer_function' );
23: wp_mail
This action hook is used to modify the email sent by WordPress. This could be used to add custom headers or modify the email content.
function custom_wp_mail_function( $wp_mail ) {
// Code to modify email
return $wp_mail;
}
add_action( 'wp_mail', 'custom_wp_mail_function' );
24: rest_api_init
This action hook is used to add custom endpoints to the WordPress REST API. This could be used to add custom functionality to the REST API.
function custom_rest_api_init_function() {
register_rest_route( 'custom', '/endpoint', array(
'methods' => 'GET',
'callback' => 'custom_rest_api_endpoint_function',
) );
}
add_action( 'rest_api_init', 'custom_rest_api_init_function' );
function custom_rest_api_endpoint_function() {
// Code to execute for custom REST API endpoint
}
25: template_include
This action hook is used to modify the template used to display a page on the front end of the website. This could be used to create custom templates for pages or posts.
function custom_template_include_function( $template ) {
if ( is_single() && get_post_type() == 'custom_post_type' ) {
$template = locate_template( array( 'single-custom_post_type.php', 'single.php' ) );
}
return $template;
}
add_filter( 'template_include', 'custom_template_include_function' );
These are just some of the many action hooks available in WordPress. Using action hooks can help you customize the functionality of your WordPress site and add custom functionality. For more information on action hooks, check out the WordPress Developer documentation on WordPress Action Hooks
If you are just starting with WordPress development, then this WordPress Developer Roadmap can help you with getting started in the WordPress developer ecosystem.
One Response
Very good articles have been posted on this site. Thanks for making such content ♥. It really helps beginners.