WordPress Filter Hooks:
As a beginner WordPress developer, understanding WordPress filter hooks is crucial for customizing your WordPress site. Filter hooks allow you to modify various aspects of your site’s output, such as post content, page titles, and more. In this article, we will discuss the most important filter hooks that every WordPress developer should know. By mastering these filter hooks, you can enhance the functionality and appearance of your WordPress site to better suit your needs.
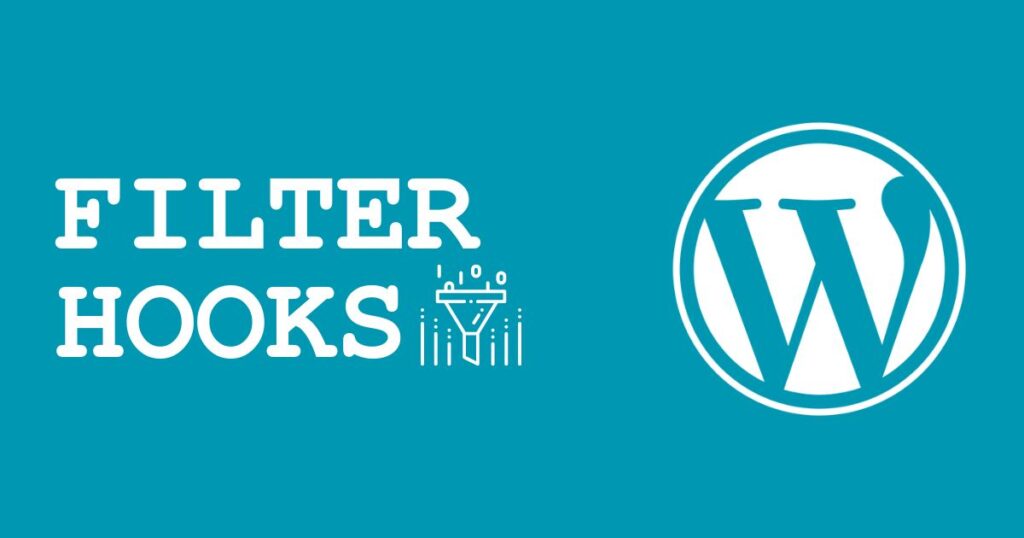
As a WordPress developer, filter hooks are an essential tool to modify the behavior of various functions and processes in WordPress. Here are some of the most important filter hooks that you should know
To use the WordPress filter hooks, you can copy these code snippets and paste them into your theme’s ‘functions.php’ file. It is better to create a child theme instead of changing directly to the main theme.
1: the_title
This filter hook is used to modify the title of a post or page. Here’s an example of how you can use this filter to modify the title of a post.
function my_custom_title_filter( $title ) {
// Add a custom prefix to the post title
$prefix = 'My Custom Prefix: ';
$new_title = $prefix . $title;
return $new_title;
}
add_filter( 'the_title', 'my_custom_title_filter' );
2: the_content
This filter hook is used to modify the content of a post or page. It is one of the most commonly used filter hooks in WordPress. Here’s an example of how you can use this filter to modify the content of a post.
function my_custom_content_filter( $content ) {
// Add a custom message to the beginning of the post content
$message = 'This is my custom message!
';
$new_content = $message . $content;
return $new_content;
}
add_filter( 'the_content', 'my_custom_content_filter' );
3: wp_nav_menu_items
This filter hook is used to modify the items in a navigation menu. Here’s an example of how you can use this filter to add a custom menu item to a navigation menu.
function my_custom_menu_item( $items, $args ) {
// Add a custom menu item to the end of the menu
$new_item = ' Custom Link ';
$items .= $new_item;
return $items;
}
add_filter( 'wp_nav_menu_items', 'my_custom_menu_item', 10, 2 );
4: the_excerpt
This filter hook is used to modify the excerpt of a post. Here’s an example of how you can use this filter to modify the excerpt of a post.
function my_custom_excerpt_filter( $excerpt ) {
// Add a custom message to the beginning of the excerpt
$message = 'This is my custom excerpt message!
';
$new_excerpt = $message . $excerpt;
return $new_excerpt;
}
add_filter( 'the_excerpt', 'my_custom_excerpt_filter' );
5: the_author
This filter hook is used to modify the author’s display name. Here’s an example of how you can use this filter to modify the author’s name.
function my_custom_author_filter( $name ) {
// Add a custom prefix to the author's name
$prefix = 'By: ';
$new_name = $prefix . $name;
return $new_name;
}
add_filter( 'the_author', 'my_custom_author_filter' );
6: the_date
This filter hook is used to modify the date display format. Here’s an example of how you can use this filter to modify the date format.
function my_custom_date_filter( $date ) {
// Change the date format
$new_date = date( 'F j, Y', strtotime( $date ) );
return $new_date;
}
add_filter( 'the_date', 'my_custom_date_filter' );
7: the_category
This filter hook is used to modify the category display for a post. Here’s an example of how you can use this filter to modify the category display.
function my_custom_category_filter( $categories ) {
// Add a custom prefix to the category display
$prefix = 'Categories: ';
$new_categories = $prefix . $categories;
return $new_categories;
}
add_filter( 'the_category', 'my_custom_category_filter' );
8: the_tags
This filter hook is used to modify the tag display for a post. Here’s an example of how you can use this filter to modify the tag display.
function my_custom_tag_filter( $tags ) {
// Add a custom prefix to the tag display
$prefix = 'Tags: ';
$new_tags = $prefix . $tags;
return $new_tags;
}
add_filter( 'the_tags', 'my_custom_tag_filter' );
9: the_permalink
This filter hook is used to modify the permalink URL for a post or page. Here’s an example of how you can use this filter to modify the permalink.
function my_custom_permalink_filter( $permalink, $post ) {
// Modify the permalink URL for a post
$new_permalink = 'http://example.com/' . $post->post_name;
return $new_permalink;
}
add_filter( 'the_permalink', 'my_custom_permalink_filter', 10, 2 );
10: the_content_more_link
This filter hook is used to modify the “Read more” link for a post. Here’s an example of how you can use this filter to modify the “Read more” link.
function my_custom_more_link_filter( $more_link_text ) {
// Change the text of the "Read more" link
$new_text = 'Continue reading...';
return $new_text;
}
add_filter( 'the_content_more_link', 'my_custom_more_link_filter' );
11: excerpt_length
This filter hook is used to modify the length of the post excerpt. Here’s an example of how you can use this filter to modify the excerpt length.
function my_custom_excerpt_length( $length ) {
// Set the excerpt length to 50 words
$new_length = 50;
return $new_length;
}
add_filter( 'excerpt_length', 'my_custom_excerpt_length' );
12: excerpt_more
This filter hook is used to modify the “Read more” link that appears at the end of the excerpt. Here’s an example of how you can use this filter to modify the “Read more” link.
function my_custom_excerpt_more( $more ) {
// Change the text of the "Read more" link
$new_more = '... Read more »';
return $new_more;
}
add_filter( 'excerpt_more', 'my_custom_excerpt_more' );
13: the_archive_title
This filter hook is used to modify the title of an archive page (e.g., category, tag, date archive). Here’s an example of how you can use this filter to modify the archive page title.
function my_custom_archive_title_filter( $title ) {
// Add a custom prefix to the archive page title
$prefix = 'Archive: ';
$new_title = $prefix . $title;
return $new_title;
}
add_filter( 'the_archive_title', 'my_custom_archive_title_filter' );
14: the_archive_description
This filter hook is used to modify the description of an archive page. Here’s an example of how you can use this filter to modify the archive page description.
function my_custom_archive_description_filter( $description ) {
// Add a custom prefix to the archive page description
$prefix = 'Description: ';
$new_description = $prefix . $description;
return $new_description;
}
add_filter( 'the_archive_description', 'my_custom_archive_description_filter' );
15: post_thumbnail_html
This filter hook is used to modify the HTML for a post thumbnail. Here’s an example of how you can use this filter to modify the post thumbnail HTML.
function my_custom_post_thumbnail_filter( $html ) {
// Add a custom class to the post thumbnail image
$new_html = str_replace( 'class="', 'class="my-custom-class ', $html );
return $new_html;
}
add_filter( 'post_thumbnail_html', 'my_custom_post_thumbnail_filter' );
16: wp_title
This filter hook is used to modify the title of a WordPress site. Here’s an example of how you can use this filter to modify the site title.
function my_custom_wp_title_filter( $title, $separator ) {
// Add a custom suffix to the site title
$suffix = 'My Custom Suffix';
$new_title = $title . $separator . $suffix;
return $new_title;
}
add_filter( 'wp_title', 'my_custom_wp_title_filter', 10, 2 );
17: login_headerurl
This filter hook is used to modify the URL for the login header logo. Here’s an example of how you can use this filter to modify the login header URL.
function my_custom_login_header_url_filter( $url ) {
// Set a custom URL for the login header logo
$new_url = 'http://example.com/';
return $new_url;
}
add_filter( 'login_headerurl', 'my_custom_login_header_url_filter' );
I hope that these WordPress filter hooks are helpful to you as a WordPress developer. By mastering these filter hooks, you can further customize your WordPress site to meet your specific needs. For more information on filter hooks, check out the WordPress Developer documentation on Filter Hooks
If you are just starting with WordPress development, then this WordPress Developer Roadmap can help you with getting started in the WordPress developer ecosystem.